Introducción
¿Te imaginas poder cambiar el estilo de un botón en todo tu sitio web con solo una clase? Los componentes reutilizables permiten justamente eso. Son fundamentales para optimizar la eficiencia, mantenibilidad y coherencia del código en proyectos web. A lo largo de los años, CSS tradicional ha sido la herramienta base para definir estilos, pero ahora Tailwind CSS, con su enfoque "utility-first", ha ganado popularidad como una alternativa rápida y eficiente para la creación de estos componentes.
El objetivo de este artículo es comparar cómo se crean componentes reutilizables utilizando CSS tradicional y Tailwind CSS, destacando las ventajas y desventajas de cada enfoque, y ofreciendo sugerencias sobre cuándo usar uno u otro en función del tipo de proyecto.
¿Qué es un componente reutilizable?
Un componente reutilizable es un bloque de código que puede ser usado múltiples veces en diferentes partes de un proyecto sin tener que volver a escribirlo. Esto facilita que tanto individuos como equipos puedan trabajar de manera más rápida y eficiente, compartiendo soluciones y manteniendo un diseño consistente en toda la aplicación.
En proyectos grandes, los componentes reutilizables permiten que los equipos colaboren de manera más eficiente, ya que reducen el código repetitivo y facilitan la actualización de estilos o funcionalidades en toda la aplicación de forma centralizada.
Breve introducción a CSS y Tailwind CSS en Nextjs
CSS tradicional:
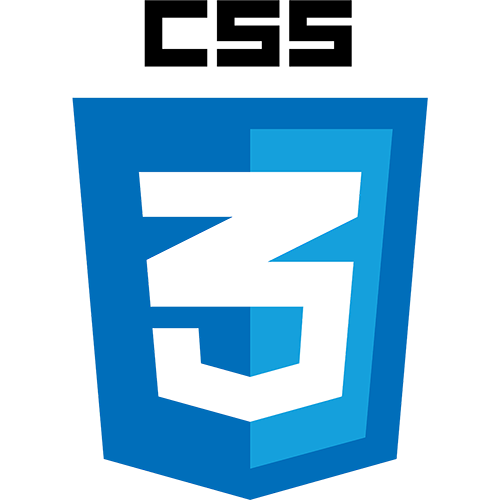
- En Next.js, el CSS tradicional se escribe en archivos .css y también puede usar CSS Modules, que limitan los estilos a componentes específicos. Los estilos se aplican mediante clases globales, IDs o selectores, y deben ser organizados manualmente por los desarrolladores.
Tailwind CSS:
.png)
- En Next.js, Tailwind CSS mantiene su enfoque "utility-first", permitiendo usar clases predefinidas directamente en los archivos JSX. Para integrarlo, es necesario configurar algunos archivos, como tailwind.config.js, específicos para su uso en Next.js.
Comparación de creación de componentes
En esta sección, exploraremos cómo se crean componentes reutilizables utilizando CSS tradicional y Tailwind CSS. Compararemos ambos enfoques a través de ejemplos prácticos, como la creación de botones y tarjetas de producto. Veremos cómo cada método gestiona la estilización, la organización del código, y cómo estos componentes pueden aplicarse de manera eficiente en diferentes áreas de un proyecto web.
1. Botón básico
Primero, vamos a ver cómo crear un botón usando CSS tradicional. Este método implica definir los estilos en un archivo CSS separado, y luego aplicar la clase al botón en el HTML.
Ejemplo de código (CSS tradicional):
En este enfoque, definimos todos los estilos en el archivo CSS (styles.css
) y luego aplicamos la clase .btn-basic
al botón. Así, cada vez que necesites este botón, solo tienes que añadir la clase en el HTML.
Ejemplo de código (Tailwind CSS):
Ahora, veamos cómo crear el mismo botón utilizando Tailwind CSS, que emplea clases de utilidad directamente en el HTML para aplicar estilos.
El botón en ambos casos se ve igual porque, aunque los códigos se aplican de manera diferente, los estilos definidos son equivalentes, lo que resulta en el mismo aspecto visual.
%25204.39.38%25E2%2580%25AFp.m..png)
Botones reutilizables en CSS
Ahora les mostraré cómo crear un componente de botón reutilizable en React usando solo CSS. Esto nos permite personalizar fácilmente el botón con distintos estilos y tamaños, manteniendo un código limpio y modular. Pasemos a la demostración.
Aquí están los estilos en CSS para el componente reutilizable de botón. Estos estilos definen una clase base para el botón y variaciones para cambiar el color y el tamaño.
Para poder ver el codigo completo en el siguiente link y además su funcionamiento https://codepen.io/karlacabanas01/pen/PoMLBNv
%25204.10.17%25E2%2580%25AFp.m..png)
2. Tarjeta de Producto con CSS
Imagina que quieres mostrar un producto en tu página web, con una imagen, un título, una descripción y un botón para agregar al carrito. Este conjunto de elementos se conoce como una tarjeta de producto, y con CSS podemos darle estilo para que se vea atractivo.
El siguiente componente es una tarjeta genérica que acepta varias propiedades (props
) como título, descripción, imagen y un conjunto de acciones (botones). Esto permite una gran flexibilidad para reutilizar el componente en diferentes contextos.
Props Dinámicas:
title
: El título de la tarjeta.description
: Una breve descripción del contenido.image
: URL de una imagen que aparece en la parte superior.actions
: Una lista de botones personalizados con texto, estilos y acciones.
Al usar props
, el componente se adapta a diferentes usos sin modificar su estructura.
Para revisar el codigo completo en el siguiente linkhttps://codepen.io/karlacabanas01/pen/dyxrjma
%25204.14.10%25E2%2580%25AFp.m.%2520(1).png)
3. Tarjeta de perfil
Las tarjetas de perfil presentan de forma rápida y atractiva información esencial de una persona, como su nombre, rol y enlaces a redes sociales. Son ideales para portafolios, perfiles de usuario y páginas de equipo. A continuación, veremos cómo crear una tarjeta de perfil reutilizable tanto con Tailwind CSS como con CSS tradicional.
Aquí podrás ver el código en tailwind:
https://codepen.io/karlacabanas01/pen/MWNqmpV
%25204.15.36%25E2%2580%25AFp.m..png)
Tailwind:
CSS:
Styles:
Pero ahora, veamos este mismo ejemplo de una manera más reutilizable. ¿A qué me refiero con esto? A que el mismo código pueda ser utilizado en varias partes de la página sin necesidad de duplicarlo. Para este caso, implementaremos el ejemplo utilizando CSS junto con React.
4. Carrusel de Imágenes con Miniaturas
El carrusel de imágenes con miniaturas permite mostrar una imagen principal y navegar por otras relacionadas mediante miniaturas. Es ideal para galerías de productos, portafolios y presentaciones visuales. A continuación, exploraremos cómo crear este componente usando Tailwind CSS y CSS tradicional.
Tailwind:
CSS:
Style:
Aquí puedes revisar el código completo https://codepen.io/karlacabanas01/pen/vYogmZp?editors=1000
%25204.16.34%25E2%2580%25AFp.m..png)
Mini Tienda con React y Tailwind CSS
En este ejemplo práctico, construiremos una aplicación de una tienda en línea simple que incluye un listado de productos, un carrito de compras dinámico y una barra de navegación. Usaremos React para crear componentes reutilizables y Tailwind CSS para estilizar de manera eficiente con clases de utilidad. Este proyecto muestra cómo combinar ambos enfoques para crear aplicaciones web escalables y elegantes.
Paso 1: Dividiendo la aplicación en componentes
En React, dividir la interfaz en componentes pequeños y reutilizables hace que el desarrollo sea más eficiente y escalable. Para esta aplicación, usaremos los siguientes componentes:
- Navbar: Muestra el título de la tienda y un contador de productos en el carrito.
- ProductList y ProductCard: Renderizan el listado de productos y cada tarjeta individual.
- Cart: Muestra los productos agregados al carrito.
- App: Componente principal que conecta todos los demás.
Ejemplo:
La barra de navegación (Navbar) es un componente sencillo que muestra el título de la tienda y un contador dinámico del carrito. Recibe el número de productos en el carrito como prop.
Paso 2: Listado de productos
ProductList contiene un arreglo con los datos de los productos (nombre, precio, imagen, etc.) y renderiza cada uno usando el componente ProductCard. Esto hace que el listado sea dinámico y fácil de mantener.
Además tenemos el ProductCart:
ProductCard
puede ser utilizado para cualquier producto, solo necesitas pasar diferentes props.
Paso 3: Implementando el carrito de compras
Cart muestra los productos seleccionados y permite al usuario proceder al pago. Si el carrito está vacío, se muestra un mensaje indicándolo.
Paso 4: Combinando todo en App
App utiliza useState para manejar el estado del carrito. La función addToCart se pasa como prop a ProductList para agregar productos seleccionados al carrito."
Este ejemplo es solo el comienzo. Puedes agregar nuevas funcionalidades, como eliminar productos del carrito o integrar una API real. ¡Experimenta y construye tu propia tienda en línea!
Para poder ver el código completo:https://codepen.io/karlacabanas01/pen/JjgqwPv?editors=1010
%25205.04.20%25E2%2580%25AFp.m..png)
Ventajas y desventajas
%25201.04.14%25E2%2580%25AFp.m..png)
💡Consejo: Si tu prioridad es el desarrollo rápido y quieres tener un estilo directo en el HTML, Tailwind CSS puede ser la opción ideal. Por otro lado, si prefieres un código estructurado y centralizado, el CSS tradicional puede ser más adecuado. Ambos enfoques tienen sus fortalezas, y la elección dependerá del tipo de proyecto y del estilo de trabajo que prefieras.
Cuándo usar Tailwind y cuándo CSS tradicional
- Tailwind CSS es ideal cuando buscas rapidez en el desarrollo y no quieres perder tiempo escribiendo CSS desde cero. Perfecto para prototipos o proyectos donde el tiempo es un factor clave.
- CSS tradicional es la mejor opción cuando necesitas un control detallado de los estilos o si estás trabajando en un proyecto que requiere personalización avanzada.
Conclusión
En resumen, la elección entre Tailwind CSS y CSS tradicional depende del tipo de proyecto y las necesidades del equipo de desarrollo. Ambos enfoques tienen sus fortalezas y desafíos, pero el enfoque "utility-first" de Tailwind permite crear componentes reutilizables más rápidamente, mientras que el CSS tradicional brinda un mayor control y organización.
Recursos adicionales