An In-Depth Analysis of Essential DDD Concepts to Create Clear and Robust Architectures
Overview of Layered Architecture in DDD

What is Layered Architecture?
Layered Architecture is a structured approach to software design that imposes a separation of responsibilities by organizing code into different layers. This approach allows for better maintainability, scalability, and testability by ensuring that each layer has a specific role within the system.
In Domain-Driven Design (DDD), Layered Architecture provides a way to structure applications in a way that preserves the integrity of the domain model while managing interactions with external systems.
The Four Main Layers in Traditional DDD Architecture
A DDD-based Layered Architecture generally consists of the following layers:
- Domain Layer (The Core) - It contains the business rules and the logic of the domain.
- Application Layer (Orchestration) - Manages use cases, coordinating domain operations.
- Infrastructure Layer (Technical Details) - Manages databases, APIs, messaging and persistence.
- Presentation Layer (User Interaction) - Manages the UI, API endpoints, or CLI interactions.
Each layer is designed to communicate in a structured manner, typically with dependencies flowing to the core of the domain.
Detailed Analysis of Each Layer in the DDD Architecture
Domain Layer (The Heart of the System)
La Domain Layer is the most important layer in DDD-based applications. It contains:
- Entities & Value Objects: They represent domain concepts and ensure the consistency of business logic.
- Aggregates & Repositories: They manage domain state and encapsulate persistence concerns.
- Domain Services: They handle domain logic that doesn't fit within an entity.
- Domain Events: They enable decoupled business logic by triggering actions based on domain changes.
This layer must be standalone of external frameworks and infrastructure details to keep it pure and reusable.
Application Layer
La Application Layer acts as an intermediary between the domain and the outside world, orchestrating workflows and applying business processes. Its key responsibilities include:
- Coordinate Use Cases: Managing business flows without implementing domain logic
- Manage Transactions: Ensure consistency across multiple domain operations.
- Interacting with External Systems: Communicate with APIs, databases, or other services.
This layer must be thin, delegating business logic to the domain layer and focusing only on orchestration.
Infrastructure Layer (Technical Implementation)
La Infrastructure Layer provides the technical implementation necessary to support the application. This includes:
- Persistence Mechanisms: Databases, file storage, or caching systems.
- External Integrations: APIs, messaging queues, or third-party services.
- Dependency Injection and Configuration: Managing dependencies and concerns at the framework level.
Although this layer contains necessary technical details, it must be kept separate from the domain logic.
Presentation Layer (User Interaction)
La Presentation Layer manages user interactions and communication with the outside world. It can include:
- A Web or Mobile UI: Frontends in React, Angular or Swift.
- An API Layer: REST or GraphQL endpoints.
- A Command Line Interface (CLI): For automation and scripting.
The key principle here is the Separation of responsibilities—presentation logic should not contain domain logic, but should be delegated to the application layer.
Alternative Architectural Approaches in DDD
Hexagonal Architecture (Ports and Adapters)
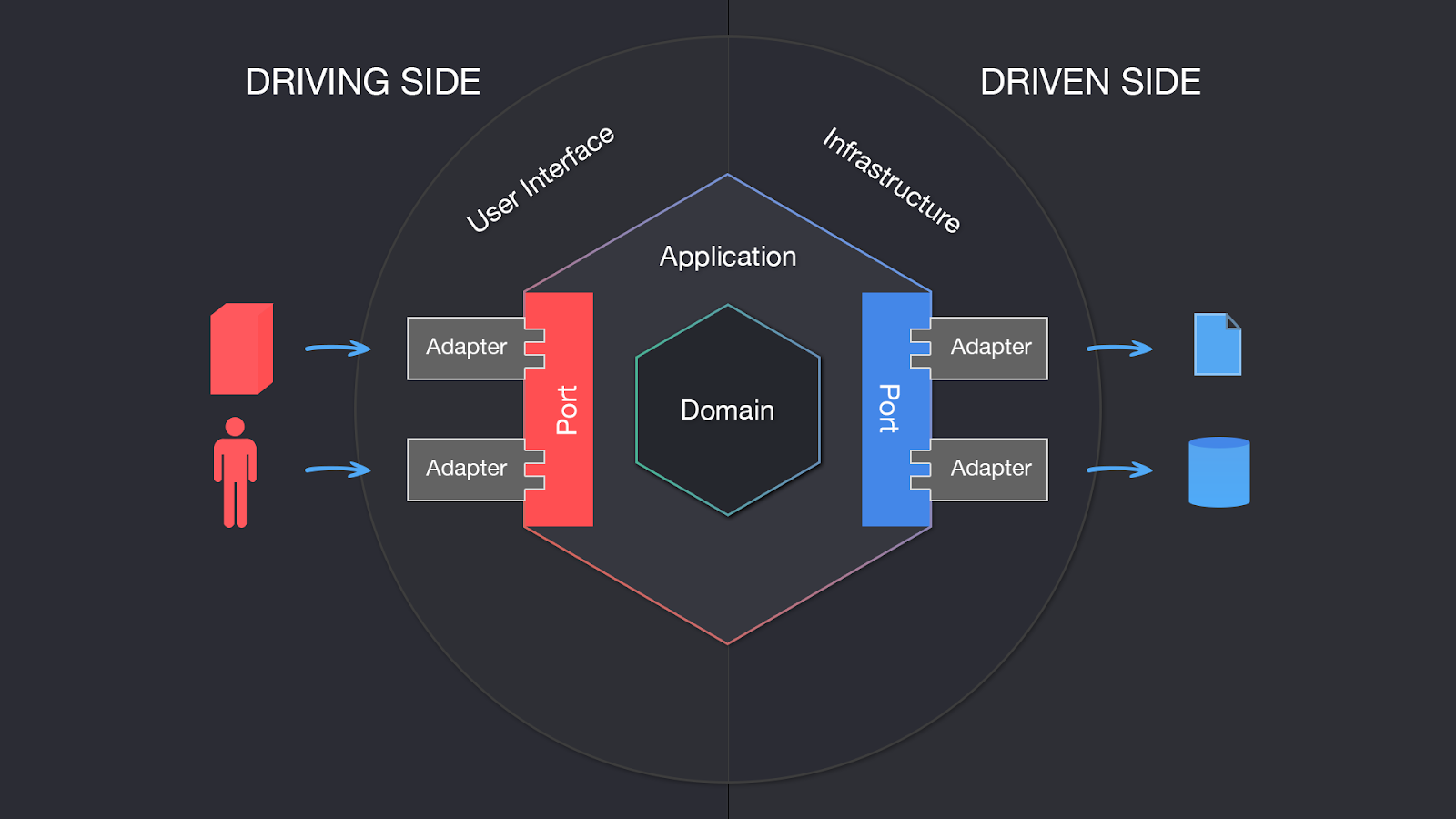
Also known as Ports and Adapters, the Hexagonal Architecture isolates the logic of the domain of external systems using Ports (interfaces) and Adapters (implementations).
- Why Use It?
- Improves testability and flexibility.
- It allows you to easily replace external dependencies (for example, to change databases).
- It encourages a clear separation between domain logic and technical concerns.
CQRS (Command Query Responsibility Segregation)
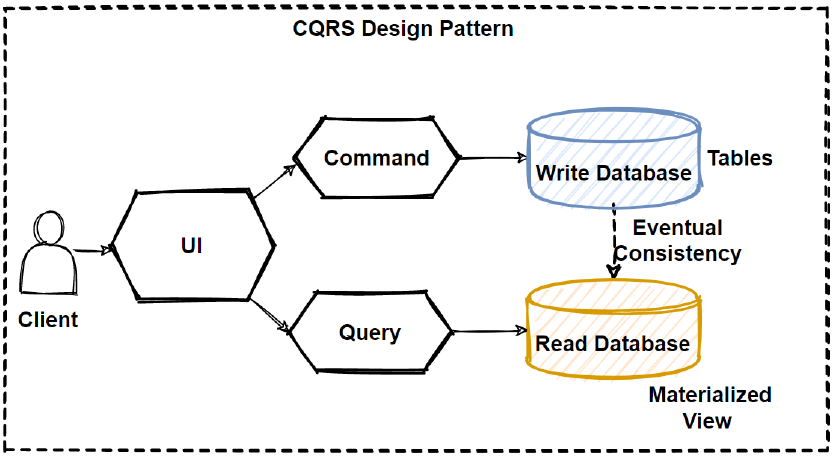
CQRS separates Commands (writing operations) of Queries (read operations), which optimizes performance and scalability.
- Why Use It?
- It improves scalability by handling reads and writes differently.
- It increases security by allowing separate permissions for reading and writing actions.
- It facilitates event sourcing, where changes are stored as a sequence of domain events.
Event-Driven Architecture & Microservices

Source:https://medium.com/@seetharamugn/the-complete-guide-to-event-driven-architecture-b25226594227
The Event-Based Architecture (EDA) allows decoupled systems that react asynchronously to domain events.
- Why Use It?
- Improves system resilience by decoupling services.
- Increase scalability by processing events in a distributed manner.
- It supports microservice architectures where each service manages its own domain.
Best Practices for Applying DDD Architecture
Keep the Domain Layer Independent
- The domain layer should not depend on external frameworks, databases, or services.
- Usa inversion of control to inject dependencies instead of directly coding them.
Use Dependency Injection to Decouple Components
- Avoid tight coupling by injecting repositories, services and adapters when necessary.
- This makes the system easier to test and adaptable to changes.
Respect Clear Boundaries Between Layers
- Keep the domain logic inside the domain layer.
- The application layer should only coordinate, not implement business rules.
- The infrastructure layer should focus on technical concerns without business logic.
Use Domain Events for Inter-Layer Communication
- Implement domain events to decouple components and improve extensibility.
- For example, when a user registers, it triggers a UserRegistered event instead of notifying other services directly.
Leverage Application Services for Use Cases, Not for Business Logic
- Application Services should only orchestrate workflows, delegating business rules to the domain layer.
- Avoid embedding domain logic in controllers or infrastructure components.
Conclusion
Domain-Driven Design offers a structured way to build scalable and maintainable applications by focusing on domain logic, while ensuring the separation of responsibilities.
- La Layered Architecture provides a clear structure but can be rigid.
- Hexagonal Architecture offers better decoupling and flexibility.
- CQRS optimizes read and write operations to improve scalability.
- Event-Driven Architecture improves the decoupling and integration of microservices.
By following best practices—such as keeping the domain layer independent, using dependency injection, and taking advantage of domain events—developers can build systems resilient, scalable and easy to maintain.
These are the next topics we'll be discussing in this Good to Great series on DDD. I hope that we will navigate this important architecture together:
- Raise code quality with Domain-Driven Design - 1/10
- Understanding Entities and Value Objects in Domain-Driven Design - 2/10
- Understanding Aggregates and Aggregates Roots in Domain-Driven Design - 3/10
- Understanding repository patterns in Domain-Driven Design - 4/10
- Understanding Domain-Services patterns in Domain-Driven Design - 5/10
- Understanding Application-Services patterns in Domain-Driven Design - 6/10
- Understand the suggested architecture pattern in Domain-Driven Design - 7/10
- Understanding Bounded Contexts in Domain-Driven Design - 8/10
- Event-Storming the modeling strategy to create Domain-Driven Design - 9/10
- Common errors and anti-patterns in Domain-Driven Design - 10/10